01 - Introduction to Intermediate Java Course
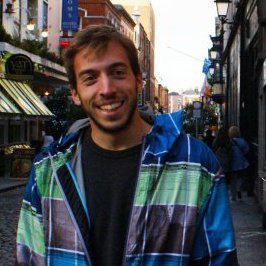
Goals
- Getting to know each other
- Get familiar with schedule, attendance, tools
- Course introduction
- Install required software
- Do some basic Java exercises (hopefully π€©)
Slides
Java Basics Recap
package com.redi.j2;
public class Main {
public static void main(String[] args) {
System.out.println("Hello World");
String name = "Memet";
String profession = "skydiver";
int age = 40;
boolean smoking = false;
someMethod(profession);
for (int i = 0; i < 10; i++) {
System.out.println("hey " + i);
}
}
private static void someMethod(String profession) {
if (profession.equals("skydiver")) {
System.out.println("Cool");
} else {
System.out.println("Not cool");
}
}
}
Exercises
Same exercises as on the slides, copied here for convenience.
Exercise 1
Write the method squares that for X and Y given by arguments prints the square of each number between X and Y.
square(1,3)
> 1 - 1
> 2 - 4
> 3 - 9
square(5,6)
> 5 - 25
> 6 - 36
Exercise 2
In german, nouns ending with e are almost always feminine. Write a method isFeminineNoun that checks if the provided word ends with e or not.
isFeminineNoun("Katze")
> true
isFeminineNoun("Hund")
> false
Bonus: can you also make sure the method also catches words ending in -ung? Those are also feminine.
Exercise 3
Letβs assume a freelancer has to pay insurance and income tax. Letβs assume insurance is fixed at 300β¬, while income tax is 9% for incomes of less than 1000β¬ (after insurance payment), and 21% otherwise.
inPocket(5000)
> 3713
inPocket(1000)
> 637
Homework
Write a program that for given value of variable βheightβ will print out the right-half of a pine tree to the console.
- The tree starts with βIβ on the top and ends with βMβ on the bottom.
- The tree is built from βXβ and βYβ characters one after another
For example, for an height
of 6 it will print:
I
XY
XYX
XYXY
XYXYX
M